This institute placed in Hardoi Up & It is the hub of Tally, CCC and O level. This is best blogger site to learn about to computer courses like:- Adca, Dca, Pgdca, CCC, O level, Dtp, Tally, Gst, Etc. You can contact us for more query on my contact no:- 9026728220, 8423606968.
Labels
- Artificial Intelligence (2)
- CCC EXAM NOTES (52)
- computer ki important jankariyan (55)
- Computer Notes (23)
- COMPUTER TIPS (28)
- computer tricks (38)
- CSS O LEVEL (43)
- Gst (1)
- HTML (2)
- IOT (3)
- IOT MCQs (1)
- IT Tools (21)
- Javascript (4)
- Libreoffice (12)
- M2R5 (O level) CSS (32)
- mobile tricks (10)
- O level (63)
- O level Notes (59)
- O level Practical (2)
- O level Project (44)
- PDF Tricks (2)
- Python program (22)
- Python Program List (3)
- ROCHAK JANKARIYA (39)
- Tally Gst (1)
- Tricks & Tips (20)
- Tricks and Tips (17)
- Viva O (1)
- Viva O Level (1)
- What is ? (1)
- Windows Tricks (11)
Information & Technology Computer Institution's
In front of Gandhi Bhawan, Numaish Chauraha Hardoi.
☎ +91 9026728220, +91 8423606968
Please Subscribe My YouTube Channel
Tuesday, February 06, 2024
Reverse Number in Python with Slicing #Howto #Youtube #ITCIComputerHardo...
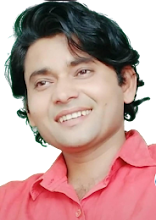.png)
Wednesday, November 01, 2023
How To Create A Text Editor Such as Notepad with Python
How To Create A Text Editor Such as Notepad with Python
import tkinter
import os
from tkinter import *
from tkinter.messagebox import *
from tkinter.filedialog import *
class Notepad:
__root = Tk()
# default window width and height
__thisWidth = 500
__thisHeight = 500
__thisTextArea = Text(__root)
__thisMenuBar = Menu(__root)
__thisFileMenu = Menu(__thisMenuBar, tearoff=0)
__thisEditMenu = Menu(__thisMenuBar, tearoff=0)
__thisHelpMenu = Menu(__thisMenuBar, tearoff=0)
# To add scrollbar
__thisScrollBar = Scrollbar(__thisTextArea)
__file = None
def __init__(self, **kwargs):
# Set icon
try:
self.__root.wm_iconbitmap("Notepad.ico")
except:
pass
# Set window size (the default is 300x300)
try:
self.__thisWidth = kwargs['width']
except KeyError:
pass
try:
self.__thisHeight = kwargs['height']
except KeyError:
pass
# Set the window text
self.__root.title("Untitled - Notepad")
# Center the window
screenWidth = self.__root.winfo_screenwidth()
screenHeight = self.__root.winfo_screenheight()
# For left-alling
left = (screenWidth / 2) - (self.__thisWidth / 2)
# For right-allign
top = (screenHeight / 2) - (self.__thisHeight / 2)
# For top and bottom
self.__root.geometry('%dx%d+%d+%d' % (self.__thisWidth,
self.__thisHeight,
left, top))
# To make the textarea auto resizable
self.__root.grid_rowconfigure(0, weight=1)
self.__root.grid_columnconfigure(0, weight=1)
# Add controls (widget)
self.__thisTextArea.grid(sticky=N + E + S + W)
# To open new file
self.__thisFileMenu.add_command(label="New",
command=self.__newFile)
# To open a already existing file
self.__thisFileMenu.add_command(label="Open",
command=self.__openFile)
# To save current file
self.__thisFileMenu.add_command(label="Save",
command=self.__saveFile)
# To create a line in the dialog
self.__thisFileMenu.add_separator()
self.__thisFileMenu.add_command(label="Exit",
command=self.__quitApplication)
self.__thisMenuBar.add_cascade(label="File",
menu=self.__thisFileMenu)
# To give a feature of cut
self.__thisEditMenu.add_command(label="Cut",
command=self.__cut)
# to give a feature of copy
self.__thisEditMenu.add_command(label="Copy",
command=self.__copy)
# To give a feature of paste
self.__thisEditMenu.add_command(label="Paste",
command=self.__paste)
# To give a feature of editing
self.__thisMenuBar.add_cascade(label="Edit",
menu=self.__thisEditMenu)
# To create a feature of description of the notepad
self.__thisHelpMenu.add_command(label="About Notepad",
command=self.__showAbout)
self.__thisMenuBar.add_cascade(label="Help",
menu=self.__thisHelpMenu)
self.__root.config(menu=self.__thisMenuBar)
self.__thisScrollBar.pack(side=RIGHT, fill=Y)
# Scrollbar will adjust automatically according to the content
self.__thisScrollBar.config(command=self.__thisTextArea.yview)
self.__thisTextArea.config(yscrollcommand=self.__thisScrollBar.set)
def __quitApplication(self):
self.__root.destroy()
# exit()
def __showAbout(self):
showinfo("Notepad", "This is a simple Notepad editor created with Python with Tkinter. This editor can create new file, edit file ,cut,copy and paste features will be available ")
def __openFile(self):
self.__file = askopenfilename(defaultextension=".txt",
filetypes=[("All Files", "*.*"),
("Text Documents", "*.txt")])
if self.__file == "":
# no file to open
self.__file = None
else:
# Try to open the file
# set the window title
self.__root.title(os.path.basename(self.__file) + " - Notepad")
self.__thisTextArea.delete(1.0, END)
file = open(self.__file, "r")
self.__thisTextArea.insert(1.0, file.read())
file.close()
def __newFile(self):
self.__root.title("Untitled - Notepad")
self.__file = None
self.__thisTextArea.delete(1.0, END)
def __saveFile(self):
if self.__file == None:
# Save as new file
self.__file = asksaveasfilename(initialfile='Untitled.txt',
defaultextension=".txt",
filetypes=[("All Files", "*.*"),
("Text Documents", "*.txt")])
if self.__file == "":
self.__file = None
else:
# Try to save the file
file = open(self.__file, "w")
file.write(self.__thisTextArea.get(1.0, END))
file.close()
# Change the window title
self.__root.title(os.path.basename(self.__file) + " - Notepad")
else:
file = open(self.__file, "w")
file.write(self.__thisTextArea.get(1.0, END))
file.close()
def __cut(self):
self.__thisTextArea.event_generate("<<Cut>>")
def __copy(self):
self.__thisTextArea.event_generate("<<Copy>>")
def __paste(self):
self.__thisTextArea.event_generate("<<Paste>>")
def run(self):
# Run main application
self.__root.mainloop()
notepad = Notepad(width=800,height=500)
notepad.run()
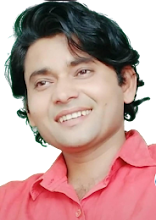.png)
Saturday, April 22, 2023
Calculation in Python (Add, Sub, Multi, Division, Square, and Module) IT...
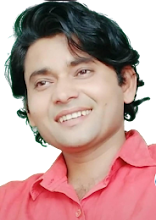.png)
Thursday, March 16, 2023
Python Program : List Method
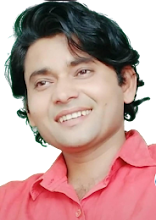.png)
Tuesday, March 14, 2023
Quiz Program With Python Programming
Create a Quiz Program With Python:-
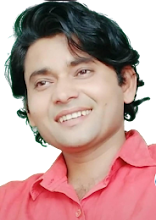.png)
How to Draw Square with Python.
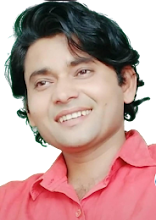.png)
How To Make Vote Age Calculator with Python
How To Make Vote Age Calculator with Python
Watch full video about this blog.
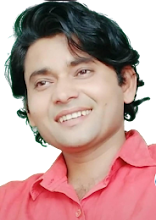.png)
Sunday, March 12, 2023
How to draw Batsman with Python Programming:-
How to draw Batsman with Python Programming:-
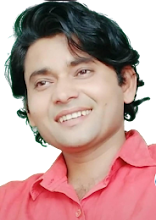.png)
How to Draw Doraemon Picture with Python Programming
How to Draw Doraemon Picture with Python Programming
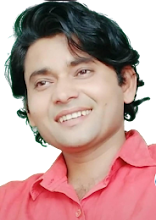.png)
Aadhar No Validation Program In python (Chech Aadhar No Is Valid or Not.)
Aadhar No Validation Program In python (Chech Aadhar No Is Valid or Not.)
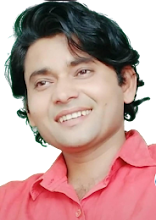.png)
Friday, February 10, 2023
For access list items/values using for loop and while loop.
Python for Loop
Flowchart of Python for Loop
Python for Loop with Python range()
Python while Loop
Flowchart of Python while Loop
Infinite while Loop with else in Python
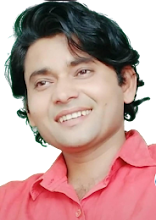.png)
Tuesday, February 07, 2023
Python program for to check if year is a leap year or not.
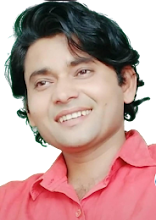.png)
Python IDLE startup failure problem solve
Python IDLE startup failure problem solve
दोस्तों अगर आप पाइथन
यूजर है और याद पाइथन प्रोग्राम को ओपन करते समय Python IDLE startup failure
प्रॉब्लम आ रही है तो यह वीडियो आपके लिए बहुत ही जरूरी है। आप वीडियो को पूरा
देखें और आप इस समस्या को मिनट में दूर कर सकते हैं, यादी
वीडियो ब्लर दिखा तो आप सेटिंग आइकन पर क्लिक करके उसकी क्वालिटी 720p या फिर 1080p चेंज कर दे वीडियो साफ दिखाएगा।
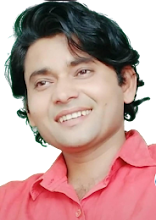.png)
Tuesday, January 31, 2023
IOT (Internet of Things) one linear Important Point
M4R5 IOT (Internet of
Things)
www.itcicomputerhardoi.blogspot.com
IOT Important Point
CCC Important points Cyber Crime, Cyber Security and Virus Libre Calc point
· IOT का पूरा नाम क्या है – इन्टरनेट ऑफ़ थिंग्स (Internet Of Things)
· IOT में थिंग्स का क्या मतलब किससे है – बस्तुओं से (वह सभी बस्तुये जो ऑन और ऑफ टेक्निक का प्रयोग करती है.
· इन्टरनेट को क्लाउड भी कहा जाता है – सत्य
· IOT क्या है – नेटवर्क और सेंसर से जुडी हुई बस्तुये.
· आई ओ टी की खोज किसने की – केविन आस्थन ने 1999 में
· IOT उपकरण डेटा को कलेक्ट करने व शेयर करने के लिए किसका उपयोग करते है – इन्टरनेट का
· IOT में किसका होना अनिवार्य है – माइक्रो कंट्रोलर
· IOT में किस टेक्नोलॉजी का प्रयोग किया जाता है- वायरलेस तकनीक का
· क्या इन्टरनेट ऑफ़ थिंग्स पूरी तरह से सेफ है – नहीं
· IOT के प्लेटफॉर्म कौन से है – AWS, माइक्रोसॉफ्ट अजुरे, सेल्सफ़ोर्स, आदि.
· IOT के फंडामेंटल कॉम्पोनेन्ट कौन से है – सेंसर, कनेक्टिविटी, डाटा प्रोसेसिंग और यूजर इंटरफ़ेस.
· IOT में वायरलेस कनेक्शन के लिए किस लेयर का प्रयोग किया जाता है – डाटा link लेयर
· IOT में पुश बटन क्या है – डिजिटल सेंसर
· IOT में किस लाइट सेंसर किस प्रकार का सेंसर है – एनालॉग सेंसर
· भौतिक दुनिया से डाटा को कैप्चर करने के लिए किस प्रकार की डिवाइस का प्रयोग किया जाता है – IOT डिवाइस का
·
Arduino Programming में सिंगल line कमेंट के लिए क्या करते है - //comment//
· Respberry क्या है – एक प्रकार का कंप्यूटर
· Respberry कंप्यूटर में किस टेक्नोलॉजी का प्रयोग किया जाता है- ऑन बोर्ड wifi व ब्लूटूथ जैसी तकनीक का
·
IOT उपकरण में
कौन –कौन से सेंसर प्रयोग किये जाते है- स्मोक सेंसर(धुआं सेंसर), प्रेसर या
दाब सेंसर, टेम्परेचर या तापमान सेंसर, Motion डिटेक्शन सेंसर, प्रोक्सिमिटी सेंसर,BMP-280,DHT-11
आदि.
· IOT में PWM क्या है – Pulse Width Modulation.
· PWM का क्या कार्य है – DC मोटर की स्पीड व डिमिंग लाइट को कण्ट्रोल करना
· बोट नेट किस प्रकार के अटैक को लांच करता है – DDos
· Arduino Programming में मोडुलो को कण्ट्रोल करने के लिए किस सिंबल का प्रयोग करते है - % (परसेंट का)
·
Arduino UNO प्रोटोटाइप बोर्ड में किस माइक्रो कंट्रोलर
का प्रयोग किया जाता है – ATmega328P
·
ATmega328P में p
का क्या मतलब है – pico-power
· Arduino Programming का डिफ़ॉल्ट मेथड क्या है - setup() और loop()
·
जब स्केच स्टार्ट होता है
तब कौन सा फंक्शन कॉल होता है – setup()
·
वेरिएबल को इनिशियलाइज़ करने
के लिए कौन सा फंक्शन है - setup()
·
Arduino
board के रिसेट होने या पॉवर अप
होने के बाद setup() फंक्शन कितनी बार चलता है – सिर्फ 1 बार
·
Arduino बोर्ड को सक्रिय रूप से नियंत्रित करने के लिए किसका उपयोग
करते है । - loop() फंक्शन
· सबसे highest डाटा रेट कम्युनिकेशन किसमें होता है – ऑप्टिकल फाइबर केबल में.
· Arduino का डिफ़ॉल्ट बूट लोडर क्या है – Optiboot
· Arduino IDE प्रोग्राम किस सॉफ्टवेर पर लिखे व अपलोड किये जाते है- स्केच (skech) पर
· Arduino में बूट लोडर को re-program करने के लिए किसका प्रयोग करते है – ICSP (In Circuit Serial Programming).
· ICSP (In Circuit Serial Programming), यह फर्मवेयर प्रोग्राम करने के लिए एक प्रोग्रामिंग विधि है- सत्य
· Arduino डिजाईन क्या है – एक प्रकार का ओपन सोर्स सॉफ्टवेर, जो एनालॉग और डिजिटल दोनों डाटा को पढ़ सकता है.
· Arduino डिजाईन सॉफ्टवेर के पास कोई ऑपरेटिंग सिस्टम नही होता है क्योंकि फर्मवेयर सॉफ्टवेर इसमें पहले से प्रोग्राम किया होता है – सत्य
· LED लाइट को कण्ट्रोल करने के लिए किस मोडूल का प्रयोग किया जाता है – Blootooth Module HC-05 का (जो स्मार्ट फ़ोन पर blootooth सिग्नल सेंड करता है)
·
IOT प्लेटफॉर्म किससे
जुड़ा है- सेंसर और डिवाइस से
·
IOT प्लेटफॉर्म
हार्डवेयर और सॉफ्टवेर को प्रोटोकॉल के द्वारा कण्ट्रोल करता है- सत्य
·
IOT में आउटपुट के लिए
किसका प्रयोग किया जाता है – Actuator का
·
किसी भी कार्य को कण्ट्रोल
करने के लिए माइक्रोकंट्रोलर और माइक्रो प्रोसेसर का प्रयोग किया जाता है – सत्य
·
अलेक्सा क्या है- एक
Voice कंट्रोलर
·
BMP-280, DHT-11,
Photoresistor क्या है – सेंसर
·
IOT में Actuator कौन से है
– स्टॉपर मोटर,फैन, LED, etc.
·
IOT में Actuator का क्या
कार्य है – इलेक्ट्रॉनिक सिग्नल को भौतिक सिग्नल में बदलने के लिए
·
IOT में Actuator को ऑपरेट
होने के लिए किस सिग्नल की आवश्यकता होती है- एनालॉग सिग्नल
·
COAP कौन सी लेयर है – सर्विस
लेयर
·
Ardunio कितने प्रकार के है
– 8 (आठ)
·
I2C क्या है – Inter Integrated Communication (यह एक प्रोटोकॉल है)
·
IOT बेस्ड पहली डिवाइस कौन
सी है – ATM
·
SCTP किस लेयर पर कार्य
करता है – Transport Layer
·
Port Address, पता होस्ट पर
एक प्रक्रिया की पहचान करता है – सत्य
·
ट्रांसपोर्ट लेयर का कार्य
क्या है – मल्टीप्लेक्सिंग और डी मल्टीप्लेक्सिंग
·
ट्रांसपोर्ट लेयर किस रूप
में डाटा प्राप्त करती है – बाइट स्ट्रीम के रूप में.
·
UDP कनेक्शन लेस प्रोटोकॉल
है – सत्य
·
UDP का पूरा नाम क्या है – User Datagram Protocol
·
UDP क्या है – एक प्रोटोकॉल
·
TCP क्या है ?- यह कनेक्शन ओरिएंटेड प्रोटोकॉल है जिसका पूरा नाम
ट्रांसमिशन कण्ट्रोल प्रोटोकॉल है.
·
IOT के लिए सबसे बेस्ट IP
वर्जन है – IPV6
·
स्मार्ट grid आर्किटेक्चर
में क्लाउड की क्या भूमिका है – डाटा को मैनेज करना.
·
पहली नई शब्दावली Arduino प्रोग्राम है जिसे "स्केच" कहा जाता है। - सत्य
· Arduino प्रोग्राम को तीन मुख्य भागों में विभाजित किया जा सकता है- Structure, Values (variables and constants), and Functions.
·
Arduino में डाटा
टाइप कौन से है - void, Boolean, char, Unsigned char, byte, int, Unsigned int, word, long, Unsigned long, short, float, double, array, String-char array, String-object
·
Arduino प्रोग्रामिंग में फंक्शन को डिक्लेअर करने के लिए कौन सा
कीवर्ड है – void
·
बूलियन डाटा दो प्रकार के
मान रखता है सत्य या असत्य था प्रत्येक बूलियन वेरिएबल के लिए 1 बाइट जगह लेता है – सत्य
• Z-wave क्या है – एक वायरलेस कम्युनिकेशन प्रोटोकॉल जिसका प्रयोग स्मार्ट होम नेटवर्किंग स्मार्ट डिवाइस को कनेक्ट करने में किया जाता है.
• Zig-Bee क्या है – एक low power, low डाटा रेट वाला वायरलेस नेटवर्क.
• Zig-Bee किस पर आधारित है – इलेक्ट्रिकल और इलेक्ट्रॉनिक्स इंजीनियर्स (IEEE) 802.15.4 मानक पर आधारित
• BLE क्या है – Blutooth Low Energy नेटवर्क जो पर्सनल एरिया नेटवर्क के अन्तेर्गत आता है.
• Respberry क्या है – एक प्रकार का कंप्यूटर
• Respberry कंप्यूटर में किस टेक्नोलॉजी का प्रयोग किया जाता है- ऑन बोर्ड wifi व ब्लूटूथ जैसी तकनीक का
• IOT उपकरण में कौन –कौन से सेंसर प्रयोग किये जाते है- स्मोक सेंसर(धुआं सेंसर), प्रेसर या दाब सेंसर, टेम्परेचर या तापमान सेंसर, Motion डिटेक्शन सेंसर, प्रोक्सिमिटी सेंसर,BMP-280,DHT-11 आदि.
• IOT में PWM क्या है – Pulse Width Modulation.
• PWM का क्या कार्य है – DC मोटर की स्पीड व डिमिंग लाइट को कण्ट्रोल करना
• बोट नेट किस प्रकार के अटैक को लांच करता है – DDos
• Arduino Programming में मोडुलो को कण्ट्रोल करने के लिए किस सिंबल का प्रयोग करते है - % (परसेंट का)
• Arduino UNO प्रोटोटाइप बोर्ड में किस माइक्रो कंट्रोलर का प्रयोग किया जाता है – ATmega328P
• ATmega328P में p का क्या मतलब है – pico-power
• Arduino Programming का डिफ़ॉल्ट मेथड क्या है - setup() और loop()
• सबसे highest डाटा रेट कम्युनिकेशन किसमें होता है – ऑप्टिकल फाइबर केबल में.
• Arduino का डिफ़ॉल्ट बूट लोडर क्या है – Optiboot
• Arduino IDE प्रोग्राम किस सॉफ्टवेर पर लिखे व अपलोड किये जाते है- स्केच (skech) पर
• Arduino में बूट लोडर को re-program करने के लिए किसका प्रयोग करते है – ICSP (In Circuit Serial Programming).
• ICSP (In Circuit Serial Programming), यह फर्मवेयर प्रोग्राम करने के लिए एक प्रोग्रामिंग विधि है- सत्य
• Arduino डिजाईन क्या है – एक प्रकार का ओपन सोर्स सॉफ्टवेर, जो एनालॉग और डिजिटल दोनों डाटा को पढ़ सकता है.
• Arduino डिजाईन सॉफ्टवेर के पास कोई ऑपरेटिंग सिस्टम नही होता है क्योंकि फर्मवेयर सॉफ्टवेर इसमें पहले से प्रोग्राम किया होता है – सत्य
• LED लाइट को कण्ट्रोल करने के लिए किस मोडूल का प्रयोग किया जाता है – Blootooth Module HC-05 का (जो स्मार्ट फ़ोन पर blootooth सिग्नल सेंड करता है)
• IOT प्लेटफॉर्म किससे जुड़ा है- सेंसर और डिवाइस से
• IOT प्लेटफॉर्म हार्डवेयर और सॉफ्टवेर को प्रोटोकॉल के द्वारा कण्ट्रोल करता है- सत्य
• IOT में आउटपुट के लिए किसका प्रयोग किया जाता है – Actuator का
• IETF का पूरा नाम क्या है – Internet Engineering Task Force
• AMQP क्या है – Advanced Message Queuing Protocol
• CoAP का पूरा नाम क्या है –Constrained Application Protocol
• LoRaWAN का पूरा नाम:- Long Range Wide Area Network (यह लाखो कम शक्ति वाले उपकरणों के साथ स्मार्ट शहरों के लिए Wan प्रोटोकॉल है.
• GS1 क्या है – Global Standards One
• O-DF क्या है – Open Data Format
• NFC क्या है – Near Field Communication
एनएफसी में इलेक्ट्रॉनिक उपकरणों के लिए संचार प्रोटोकॉल होते हैं, आमतौर पर एक मोबाइल डिवाइस और एक मानक डिवाइस का प्रयोग करता है।
• RFID क्या है – रेडियो फ्रीक्वेंसी आइडेंटिफिकेशन
RFID तकनीक वस्तुओं से जुड़े टैग को पहचानने और ट्रैक करने के लिए तथा 2-वे रेडियो ट्रांसमीटर-रिसीवर का उपयोग करती है
• Low-Energy Wireless (LEW) - यह तकनीक एक IoT सिस्टम के सबसे अधिक शक्ति वाले पहलू की जगह लेती है। हालांकि सेंसर और अन्य तत्व लंबे समय तक बंद हो सकते हैं।
• रेडियो प्रोटोकॉल - ZigBee, Z-Wave, and Thread are radio protocols for creating low-rate private area networks.
• Types of Sensor:- accelerometers, temperature sensors, magnetometers, proximity sensors, gyroscopes, image sensors, acoustic sensors, light sensors, pressure sensors, gas RFID sensors, humidity sensors , micro flow sensors
• 6LoWPAN - यह लो-पावर वायरलेस पर्सनल एरिया नेटवर्क पर IPv6 के लिए है। यह सीमित संसाधनों वाले उपकरणों द्वारा आवश्यक कम डेटा दर वायरलेस का समर्थन करने के लिए संपीड़न तकनीक प्रदान करता है।
• RPL- यह कम-शक्ति और हानिपूर्ण नेटवर्क) के लिए यह डिस्टेंस वेक्टर आईपीवी 6 प्रोटोकॉल है जो विभिन्न क्षमता वाले उपकरणों के जटिल नेटवर्क में सर्वोत्तम संभव पथ खोजने की अनुमति देता है।
• LLNs क्या है - Low-Power And Lossy Networks
• IoT सिस्टम के बिल्डिंग ब्लाक में क्या आवश्यक है - चार चीजें ( सेंसर, प्रोसेसर, गेटवे, एप्लिकेशन)
सेंसर:- ये IoT उपकरणों का फ्रंट एंड बनाते हैं। उनका मुख्य उद्देश्य वे रीयल-टाइम डेटा एकत्र करना या इसके आसपास (एक्ट्यूएटर्स) को डेटा देना है।
प्रोसेसर:- यह IoT सिस्टम का दिमाग हैं, यह डेटा को इंटेलिजेंस देता है। उनका मुख्य कार्य सेंसर द्वारा कैप्चर किए गए डेटा को संसाधित करना ताकि भारी मात्रा में एकत्र किए गए कच्चे डेटा से मूल्यवान डेटा निकाला जा सके। प्रोसेसर ज्यादातर रीयल-टाइम आधार पर काम करते हैं और इन्हें एप्लिकेशन द्वारा आसानी से नियंत्रित किया जा सकता है। ये डेटा को सुरक्षित(एन्क्रिप्शन और डिक्रिप्शन) करने के लिए भी जिम्मेदार हैं.
गेटवे:- गेटवे डेटा के संचार में मदद करता है। यह संसाधित डेटा को रूट करने उचित उपयोग के लिए उचित स्थानों पर भेजने के लिए जिम्मेदार हैं। यह डेटा को नेटवर्क कनेक्टिविटी प्रदान करता है। LAN, WAN, PAN, आदि नेटवर्क गेटवे के उदाहरण हैं।
एप्लिकेशन:- यह IoT सिस्टम का दूसरा एंड बनाते हैं। एकत्र किए गए सभी डेटा के समुचित उपयोग के लिए application आवश्यक हैं। एप्लिकेशन उपयोगकर्ताओं द्वारा नियंत्रित होते, जिसके उदाहरन: होम ऑटोमेशन ऐप, सुरक्षा प्रणाली, औद्योगिक नियंत्रण केंद्र आदि हैं।
Python project and practical O level web desigining paper point
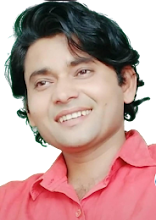.png)